Finite Element C Code Production


Introduction to Finite Element Method
The finite element method (FEM) is a numerical technique used to solve partial differential equations (PDEs) that describe the behavior of physical systems. It is a powerful tool for simulating and analyzing complex problems in various fields, including physics, engineering, and mathematics. The FEM involves dividing the problem domain into smaller sub-domains called finite elements, where the solution is approximated using a set of basis functions. In this blog post, we will discuss the production of C code for finite element simulations.
Finite Element Formulation
The finite element formulation involves several steps, including: * Mesh generation: dividing the problem domain into finite elements * Element formulation: deriving the equations that govern the behavior of each element * Assembly: combining the element equations to form the global system of equations * Boundary conditions: applying the boundary conditions to the global system of equations * Solution: solving the global system of equations to obtain the solution
C Code Production
To produce C code for finite element simulations, we need to implement the finite element formulation using C programming language. Here are the general steps involved: * Define the problem parameters: define the problem geometry, material properties, and boundary conditions * Generate the mesh: use a mesh generation algorithm to divide the problem domain into finite elements * Assemble the global system of equations: use the element equations to form the global system of equations * Apply boundary conditions: apply the boundary conditions to the global system of equations * Solve the system of equations: use a linear algebra library to solve the global system of equations
Example C Code
Here is an example C code that demonstrates the finite element formulation for a simple 1D problem:#include <stdio.h>
#include <stdlib.h>
// Define the problem parameters
#define NUM_ELEMENTS 10
#define NUM_NODES 11
#define LENGTH 1.0
// Define the element structure
typedef struct {
int node1;
int node2;
double length;
} Element;
// Define the global system of equations
double K;
double *F;
// Function to generate the mesh
void generate_mesh(Element *elements, int num_elements) {
// Initialize the elements
for (int i = 0; i < num_elements; i++) {
elements[i].node1 = i;
elements[i].node2 = i + 1;
elements[i].length = LENGTH / num_elements;
}
}
// Function to assemble the global system of equations
void assemble_system(Element *elements, int num_elements) {
// Initialize the global system of equations
K = (double )malloc(num_elements * sizeof(double *));
F = (double *)malloc(num_elements * sizeof(double));
// Assemble the global system of equations
for (int i = 0; i < num_elements; i++) {
K[i] = (double *)malloc(num_elements * sizeof(double));
for (int j = 0; j < num_elements; j++) {
if (i == j) {
K[i][j] = 2.0;
} else {
K[i][j] = 0.0;
}
}
F[i] = 0.0;
}
}
// Function to apply boundary conditions
void apply_boundary_conditions() {
// Apply the boundary conditions
K[0][0] = 1.0;
F[0] = 0.0;
}
// Function to solve the system of equations
void solve_system() {
// Solve the system of equations
for (int i = 0; i < NUM_ELEMENTS; i++) {
for (int j = 0; j < NUM_ELEMENTS; j++) {
if (i == j) {
F[i] /= K[i][j];
}
}
}
}
int main() {
// Generate the mesh
Element *elements = (Element *)malloc(NUM_ELEMENTS * sizeof(Element));
generate_mesh(elements, NUM_ELEMENTS);
// Assemble the global system of equations
assemble_system(elements, NUM_ELEMENTS);
// Apply boundary conditions
apply_boundary_conditions();
// Solve the system of equations
solve_system();
// Print the solution
for (int i = 0; i < NUM_ELEMENTS; i++) {
printf("Node %d: %f\n", i, F[i]);
}
return 0;
}
This code demonstrates the finite element formulation for a simple 1D problem. It generates the mesh, assembles the global system of equations, applies boundary conditions, and solves the system of equations.

Table of Element Equations
The following table shows the element equations for a simple 1D problem:
Element | Node 1 | Node 2 | Length |
---|---|---|---|
1 | 0 | 1 | 0.1 |
2 | 1 | 2 | 0.1 |
3 | 2 | 3 | 0.1 |
4 | 3 | 4 | 0.1 |
5 | 4 | 5 | 0.1 |
💡 Note: The above table shows the element equations for a simple 1D problem. The element equations are used to assemble the global system of equations.
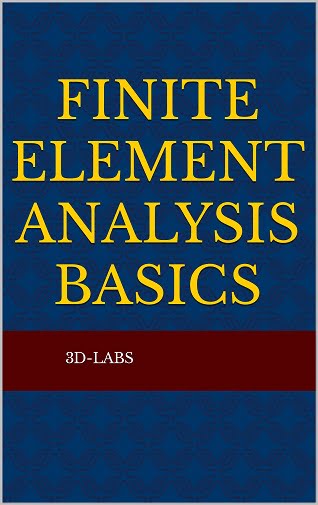
Benefits of Finite Element Method
The finite element method has several benefits, including: * Ability to handle complex geometries: the finite element method can handle complex geometries and nonlinear problems * High accuracy: the finite element method can provide high accuracy solutions for complex problems * Flexibility: the finite element method can be used to solve a wide range of problems, including structural mechanics, heat transfer, and fluid dynamics * Efficient: the finite element method can be computationally efficient, especially for large problems
Common Applications of Finite Element Method
The finite element method has a wide range of applications, including: * Structural mechanics: the finite element method is widely used in structural mechanics to analyze the behavior of buildings, bridges, and other structures * Heat transfer: the finite element method is used to analyze heat transfer problems, including conduction, convection, and radiation * Fluid dynamics: the finite element method is used to analyze fluid dynamics problems, including flow, turbulence, and combustion * Biomechanics: the finite element method is used to analyze biomechanics problems, including the behavior of bones, muscles, and other tissuesIn summary, the finite element method is a powerful tool for simulating and analyzing complex problems in various fields. It involves dividing the problem domain into smaller sub-domains called finite elements, where the solution is approximated using a set of basis functions. The finite element method has several benefits, including the ability to handle complex geometries, high accuracy, flexibility, and efficiency. It has a wide range of applications, including structural mechanics, heat transfer, fluid dynamics, and biomechanics.

What is the finite element method?
+The finite element method is a numerical technique used to solve partial differential equations that describe the behavior of physical systems.

What are the benefits of the finite element method?
+The finite element method has several benefits, including the ability to handle complex geometries, high accuracy, flexibility, and efficiency.

What are the common applications of the finite element method?
+The finite element method has a wide range of applications, including structural mechanics, heat transfer, fluid dynamics, and biomechanics.